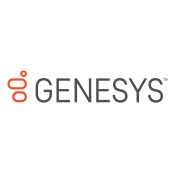
The Interaction Center Extension Library (IceLib for short) is a programming API that allows developers to create custom applications that leverage the Interaction Center to solve business problems. IceLib is for developers who use modern .Net languages, such as C# or VB.Net.
IceLib provides a clean architecture that applications can use to manage sessions with the Interaction Center (IC) server. IceLib provides session creation and login functions that allow applications to connect with one or more IC servers using the login options that are available in Interaction Client .Net Edition (e.g. user, password, station, remote number, remote station, persistent, audio enabled, etc.).
Welcome
IceLib interfaces with SessionManager, the IC subsystem that brokers connections between client applications and a given IC Server. Custom IceLib applications fully leverage SessionManager, just like internally developed IC applications. For example, Interaction Desktop, Interaction Client Web Edition, Interaction Fax, Interaction VoiceMail, Interaction Client .Net Edition, and Interaction Client Outlook Edition all use IceLib and take advantage of its SessionManager capability. IceLib is feature-license based, not per-seat or per-session. Client sessions require client licenses.
Generally speaking, IceLib provides the means to work with Interactions, Directories, People, Interaction Tracker, and Unified Messaging. It manages connections with the IC server, specifies authentication and station settings, watches for connection state-change events, and performs actions relative to the connected Session user.
What's New in IceLib
IceLib API
IceLib follows the direction that Microsoft has taken with public APIs. It conforms with Microsoft's design guidance and best practices for public APIs and framework development. It utilizes familiar style and naming conventions. A supported version of Microsoft .NET Framework should be installed on the system which utilizes Icelib.
For example, IceLib is object-based, rather than interface-based. This reflects the direction that Microsoft and the .NET technologies have taken to go beyond COM, in accordance with the .NET Framework Design Guidelines.
IceLib conforms with the Common Language Specification (CLS), a standard that defines naming restrictions, data types, and rules to which assemblies must conform if they are to be used across programming languages. This means that IceLib is compatible with C#, VB.Net, and all other .Net languages.
IceLib is a strongly typed API. Common attributes are accessible as properties, enumerations, or constants. IceLib supports generics, events, asynchronous patterns, and nullable types. Properties are used instead of public fields. This helps make the API future-proof, since changes can be made to a property get/set without affecting existing third-party application usage.
IceLib provides a consistent set of stable interfaces, that expose Interaction Client .Net Edition feature sets to third-party applications. It provides a stable foundation for application development. IceLib's internal consistency promotes intuitive use, and its adherence with .Net eases understanding, reduces ramp-up time, and speeds development.
Change notification is implemented using events that follow the common Object/EventArgs pattern. This allows multiple notification recipients to be registered. It also allows recipients granular control over which notifications they receive. Developers should name the custom delegate for an event "FooEventHandler". Foo does not have to match the name of the event if FooEventHandler is generally useful for multiple events in the system. FooEventHandler's first parameter should be "object sender" and the second parameter should either be "EventArgs e" (and set to EventArgs.Empty) if no arguments are needed, or if a custom FooEventArgs class that inherits from EventArgs (or CancelEventArgs).
Consistent API design promotes intuitive use by application developers. Further, it can reduce the number of bugs when correct usage patterns have previously been learned. The ease of use improvements gained through design consistency are sometimes termed "The Power of Sameness". This is beneficial to customers since it can reduce application development costs.
IceLib provides synchronous (blocking) and asynchronous (non-blocking) versions of most methods, especially those methods that make a server call. This convention allows the developer the convenience of choosing which programming model to use. It also allows him to switch back and forth between the two models where appropriate within the same application.
In IceLib, errors are reported via exceptions rather than by returning or querying error codes. Methods are designed to "fail fast", by evaluating parameters early and to throw meaningful argument exceptions. This helps developers to identify issues with code more quickly and easily.
Getting Started
The Getting Started section includes documentation on some key areas of the IceLib API, such as overview information and examples for common use cases. It can be challenging for a new developer to determine where to get started with the elements of a namespace. These topics are meant to "jump start" that effort, providing some important aspects to be aware of for a particular API area. Additionally, by providing focused example snippets around common tasks, these topics provide quicker understanding of what's possible and how to make those possibilities into reality. Then, the more full-featured example applications extend the core concepts and API usages into the context of developing real applications that allow users to perform larger tasks.
General Concepts
The Concepts section includes documentation on some fundamental concepts which are common to many different areas of the IceLib API. Some concepts are simple, while others contain nuances that can trip up developers. These topics strive to clarify these general points, providing conceptual context for utilizing elements throughout the IceLib API.